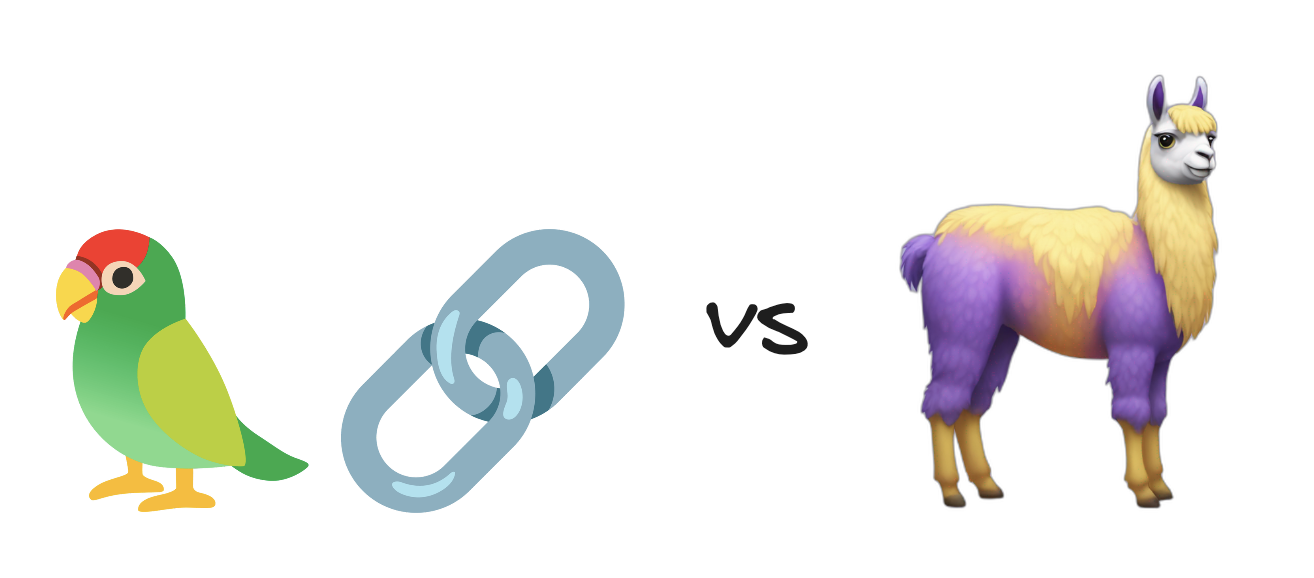
Introduction:
Big Language Fashions (LLMs) in the mean time are broadly accessible for major chatbot based totally utilization, nevertheless integrating them into additional difficult functions may very well be troublesome. Lucky for builders, there are devices that streamline the blending of LLMs to functions, two of in all probability probably the most excellent being LangChain and LlamaIndex.
These two open-source frameworks bridge the outlet between the raw power of LLMs and smart, user-ready apps – each offering a novel set of devices supporting builders of their work with LLMs. These frameworks streamline key capabilities for builders, similar to RAG workflows, data connectors, retrieval, and querying methods.
On this text, we’re going to uncover the wants, choices, and strengths of LangChain and LlamaIndex, providing steering on when each framework excels. Understanding the variations will allow you to make the suitable choice in your LLM-powered functions.
Overview of Each Framework:
LangChain
Core Aim & Philosophy:
LangChain was created to simplify the occasion of functions that rely upon big language fashions by providing abstractions and devices to assemble difficult chains of operations which will leverage LLMs efficiently. Its philosophy services spherical establishing versatile, reusable components that make it easy for builders to create intricate LLM functions without having to code every interaction from scratch. LangChain is very suited to functions requiring dialog, sequential logic, or difficult job flows that need context-aware reasoning.
Construction
LangChain’s construction is modular, with each half constructed to work independently or collectively as half of a much bigger workflow. This modular technique makes it easy to customize and scale, counting on the desires of the equipment. At its core, LangChain leverages chains, brokers, and memory to supply a flexible development which will cope with one thing from simple Q&A methods to difficult, multi-step processes.
Key Choices
Doc loaders in LangChain are pre-built loaders that current a unified interface to load and course of paperwork from fully totally different sources and codecs along with PDFs, HTML, txt, docx, csv, and so forth. As an illustration, you presumably can merely load a PDF doc using the PyPDFLoader, scrape web content material materials using the WebBaseLoader, or join with cloud storage corporations like S3. This efficiency is very useful when establishing functions that should course of plenty of data sources, similar to doc Q&A methods or data bases.
from langchain.document_loaders import PyPDFLoader, WebBaseLoader
# Loading a PDF
pdf_loader = PyPDFLoader("doc.pdf")
pdf_docs = pdf_loader.load()
# Loading web content material materials
web_loader = WebBaseLoader("https://nanonets.com")
web_docs = web_loader.load()
Textual content material splitters cope with the chunking of paperwork into manageable contextually aligned gadgets. This could be a key precursor to right RAG pipelines. LangChain affords quite a few splitting strategies for example the RecursiveCharacterTextSplitter, which splits textual content material whereas making an attempt to maintain up inter-chunk context and semantic which means. Chances are you’ll configure chunk sizes and overlap to steadiness between context preservation and token limits.
from langchain.text_splitter import RecursiveCharacterTextSplitter
splitter = RecursiveCharacterTextSplitter(
chunk_size=1000,
chunk_overlap=200,
separators=["nn", "n", " ", ""]
)
chunks = splitter.split_documents(paperwork)
Quick templates help in standardizing prompts for quite a few duties, guaranteeing consistency all through interactions. LangChain means you can define these reusable templates with variables which may be crammed dynamically, which is a robust operate for creating fixed nevertheless customizable prompts. This consistency means your software program may be easier to maintain up and substitute when important. A wonderful method to utilize inside your templates is ‘few-shot’ prompting, in several phrases, along with examples (constructive and unfavourable).
from langchain.prompts import PromptTemplate
# Define a few-shot template with constructive and unfavourable examples
template = PromptTemplate(
input_variables=["topic", "context"],
template="""Write a summary about {topic} considering this context: {context}
Examples:
### Optimistic Occasion 1:
Topic: Native climate Change
Context: Newest evaluation on the impacts of native climate change on polar ice caps
Summary: Newest analysis current that polar ice caps are melting at an accelerated cost ensuing from rising worldwide temperatures. This melting contributes to rising sea ranges and impacts ecosystems reliant on ice habitats.
### Optimistic Occasion 2:
Topic: Renewable Energy
Context: Advances in photograph voltaic panel effectivity
Summary: Enhancements in photograph voltaic know-how have led to additional setting pleasant panels, making photograph voltaic vitality a additional viable and cost-effective totally different to fossil fuels.
### Damaging Occasion 1:
Topic: Native climate Change
Context: Impacts of native climate change on polar ice caps
Summary: Native climate change is happening everywhere and has outcomes on each little factor. (This summary is imprecise and lacks component explicit to polar ice caps.)
### Damaging Occasion 2:
Topic: Renewable Energy
Context: Advances in photograph voltaic panel effectivity
Summary: Renewable vitality is sweet because of it helps the environment. (This summary is overly frequent and misses specifics about photograph voltaic panel effectivity.)
### Now, based totally on the topic and context provided, generate an in depth, explicit summary:
Topic: {topic}
Context: {context}
Summary:"""
)
# Format the instant with a model new occasion
instant = template.format(topic="AI", context="Newest developments in machine finding out")
print(instant)
LCEL represents the fashionable technique to establishing chains in LangChain, offering a declarative methodology to compose LangChain components. It’s designed for production-ready functions from the start, supporting each little factor from simple prompt-LLM mixtures to difficult multi-step chains. LCEL affords built-in streaming help for optimum time-to-first-token, computerized parallel execution of neutral steps, and full tracing by way of LangSmith. This makes it notably helpful for manufacturing deployments the place effectivity, reliability, and observability are important. As an illustration, you would presumably assemble a retrieval-augmented period (RAG) pipeline that streams outcomes as they’re processed, handles retries routinely, and affords detailed logging of each step.
from langchain.chat_models import ChatOpenAI
from langchain.prompts import ChatPromptTemplate
from langchain.schema.output_parser import StrOutputParser
# Straightforward LCEL chain
instant = ChatPromptTemplate.from_messages([
("system", "You are a helpful assistant."),
("user", "{input}")
])
chain = instant | ChatOpenAI() | StrOutputParser()
# Stream the outcomes
for chunk in chain.stream({"enter": "Inform me a story"}):
print(chunk, end="", flush=True)
Chains are one in all LangChain’s strongest choices, allowing builders to create delicate workflows by combining plenty of operations. A sequence might start with loading a doc, then summarizing it, and eventually answering questions on it. Chains are primarily created using LCEL (LangChain Execution Language). This instrument makes it easy to every assemble custom-made chains and use ready-made, off-the-shelf chains.
There are a variety of prebuilt LCEL chains accessible:
- create_stuff_document_chain: Use whilst you want to format a listing of paperwork proper right into a single instant for the LLM. Assure it matches all through the LLM’s context window as all paperwork are included.
- load_query_constructor_runnable: Generates queries by altering pure language into allowed operations. Specify a listing of operations sooner than using this chain.
- create_retrieval_chain: Passes a client inquiry to a retriever to fetch associated paperwork. These paperwork and the distinctive enter are then utilized by the LLM to generate a response.
- create_history_aware_retriever: Takes in dialog historic previous and makes use of it to generate a query, which is then handed to a retriever.
- create_sql_query_chain: Applicable for producing SQL database queries from pure language.
Legacy Chains: There are moreover plenty of chains accessible from sooner than LCEL was developed. As an illustration, SimpleSequentialChain, and LLMChain.
from langchain.chains import SimpleSequentialChain, LLMChain
from langchain.llms import OpenAI
import os
os.environ['OPENAI_API_KEY'] = "YOUR_API_KEY"
llm=OpenAI(temperature=0)
summarize_chain = LLMChain(llm=llm, instant=summarize_template)
categorize_chain = LLMChain(llm=llm, instant=categorize_template)
full_chain = SimpleSequentialChain(
chains=[summarize_chain, categorize_chain],
verbose=True
)
Brokers symbolize a additional autonomous technique to job completion in LangChain. They’ll make choices about which tools to utilize based totally on client enter and may execute multi-step plans to understand aims. Brokers can entry quite a few devices like search engines like google and yahoo like google and yahoo, calculators, or custom-made APIs, they normally can resolve recommendations on the right way to use these devices in response to client requests. As an illustration, an agent might help with evaluation by trying the net, summarizing findings, and formatting the outcomes. LangChain has plenty of types of agents along with Instrument Calling, OpenAI Devices/Options, Structured Chat, JSON Chat, ReAct, and Self Ask with Search.
from langchain.brokers import create_react_agent, Instrument
from langchain.devices import DuckDuckGoSearchRun
search = DuckDuckGoSearchRun()
devices = [
Tool(
name="Search",
func=search.run,
description="useful for searching information online"
)
]
agent = create_react_agent(devices, llm, instant)
Memory methods in LangChain enable functions to maintain up context all through interactions. This enables the creation of coherent conversational experiences or sustaining of state in long-running processes. LangChain supplies quite a few memory kinds, from simple dialog buffers to additional delicate trimming and summary-based memory methods. As an illustration, you would presumably use dialog memory to maintain up context in a buyer help chatbot, or entity memory to hint explicit particulars about prospects or topics over time.
There are numerous sorts of memory in LangChain, counting on the extent of retention and complexity:
- Main Memory Setup: For a major memory technique, messages are handed instantly into the model instant. This simple sort of memory makes use of the latest dialog historic previous as context for responses, allowing the model to answer almost about present exchanges. ‘conversationbuffermemory’ is an environment friendly occasion of this.
- Summarized Memory: For additional difficult conditions, summarized memory distills earlier conversations into concise summaries. This technique can improve effectivity by altering verbose historic previous with a single summary message, which maintains vital context with out overwhelming the model. A summary message is generated by prompting the model to condense the entire chat historic previous, which could then be updated as new interactions occur.
- Computerized Memory Administration with LangGraph: LangChain’s LangGraph permits computerized memory persistence via the usage of checkpoints to deal with message historic previous. This method permits builders to assemble chat functions that routinely keep in mind conversations over prolonged intervals. Using the MemorySaver checkpointer, LangGraph functions can protect a structured memory with out exterior intervention.
- Message Trimming: To deal with memory successfully, significantly when dealing with restricted model context, LangChain supplies the trim_messages utility. This utility permits builders to keep up solely the most recent interactions by eradicating older messages, thereby focusing the chatbot on the latest context with out overloading it.
from langchain.memory import ConversationBufferMemory
from langchain.chains import ConversationChain
memory = ConversationBufferMemory()
dialog = ConversationChain(
llm=llm,
memory=memory,
verbose=True
)
# Memory maintains context all through interactions
dialog.predict(enter="Hiya, I'm John")
dialog.predict(enter="What's my establish?") # Will keep in mind "John"
LangChain is a extraordinarily modular, versatile framework that simplifies establishing functions powered by big language fashions by way of well-structured components. With its many choices—doc loaders, customizable instant templates, and superior memory administration—LangChain permits builders to cope with difficult workflows successfully. This makes LangChain excellent for functions that require nuanced administration over interactions, job flows, or conversational state. Subsequent, we’ll research LlamaIndex to see the way in which it compares!
LlamaIndex
Core Aim & Philosophy:
LlamaIndex is a framework designed significantly for setting pleasant data indexing, retrieval, and querying to spice up interactions with big language fashions. Its core operate is to connect LLMs with unstructured data, making it easy for functions to retrieve associated information from enormous datasets. The philosophy behind LlamaIndex is centered spherical creating versatile, scalable data indexing choices that let LLMs to entry associated data on-demand, which is very useful for functions centered on doc retrieval, search, and Q&A methods.
Construction
LlamaIndex’s construction is optimized for retrieval-heavy functions, with an emphasis on data indexing, versatile querying, and setting pleasant memory administration. Its construction comprises Nodes, Retrievers, and Query Engines, each designed to cope with explicit factors of data processing. Nodes cope with data ingestion and structuring, retrievers facilitate data extraction, and query engines streamline querying workflows, all of which work in tandem to supply fast and reliable entry to saved data. LlamaIndex’s construction permits it to connect seamlessly with vector databases, enabling scalable and high-speed doc retrieval.
Key Choices
Paperwork and Nodes are data storage and structuring fashions in LlamaIndex that break down big datasets into smaller, manageable components. Nodes allow data to be listed for quick retrieval, with customizable chunking strategies for quite a few doc kinds (e.g., PDFs, HTML, or CSV data). Each Node moreover holds metadata, making it potential to filter and prioritize data based totally on context. As an illustration, a Node might retailer a chapter of a doc along with its title, creator, and topic, which helps LLMs query with bigger relevance.
from llama_index.core.schema import TextNode, Doc
from llama_index.core.node_parser import SimpleNodeParser
# Create nodes manually
text_node = TextNode(
textual content material="LlamaIndex is an data framework for LLM functions.",
metadata={"provide": "documentation", "topic": "introduction"}
)
# Create nodes from paperwork
parser = SimpleNodeParser.from_defaults()
paperwork = [
Document(text="Chapter 1: Introduction to LLMs"),
Document(text="Chapter 2: Working with Data")
]
nodes = parser.get_nodes_from_documents(paperwork)
Retrievers are liable for querying the listed data and returning associated paperwork to the LLM. LlamaIndex affords quite a few retrieval methods, along with typical keyword-based search, dense vector-based retrieval for semantic search, and hybrid retrieval that mixes every. This flexibility permits builders to choose or combine retrieval methods based totally on their software program’s desires. Retrievers may very well be built-in with vector databases like FAISS or KDB.AI for high-performance, large-scale search capabilities.
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader
from llama_index.core.retrievers import VectorIndexRetriever
# Create an index
paperwork = SimpleDirectoryReader('.').load_data()
index = VectorStoreIndex.from_documents(paperwork)
# Vector retriever
vector_retriever = VectorIndexRetriever(
index=index,
similarity_top_k=2
)
# Retrieve nodes
query = "What's LlamaIndex?"
vector_nodes = vector_retriever.retrieve(query)
print(f"Vector Outcomes: {[node.text for node in vector_nodes]}")
Query Engines act as a result of the interface between the equipment and the listed data, coping with and optimizing search queries to ship in all probability probably the most associated outcomes. They help superior querying decisions similar to key phrase search, semantic similarity search, and customised filters, allowing builders to create delicate, contextualized search experiences. Query engines are adaptable, supporting parameter tuning to refine search accuracy and relevance, and making it potential to mix LLM-driven functions instantly with data sources.
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader, Settings
from llama_index.llms.openai import OpenAI
from llama_index.core.node_parser import SentenceSplitter
import os
os.environ['OPENAI_API_KEY'] = "YOUR_API_KEY"
GENERATION_MODEL = 'gpt-4o-mini'
llm = OpenAI(model=GENERATION_MODEL)
Settings.llm = llm
# Create an index
paperwork = SimpleDirectoryReader('.').load_data()
index = VectorStoreIndex.from_documents(paperwork, transformations=[SentenceSplitter(chunk_size=2048, chunk_overlap=0)],)
query_engine = index.as_query_engine()
response = query_engine.query("What's LlamaIndex?")
print(response)
LlamaIndex supplies data connectors that let for seamless ingestion from quite a few data sources, along with databases, file methods, and cloud storage. Connectors cope with data extraction, processing, and chunking, enabling functions to work with big, difficult datasets with out information formatting. That’s significantly helpful for functions requiring multi-source data fusion, like data bases or in depth doc repositories.
Totally different specialised data connectors may be discovered on LlamaHub, a centralized repository all through the LlamaIndex framework. These are prebuilt connectors inside a unified and fixed interface that builders can use to mix and pull in data from quite a few sources. By way of the usage of LlamaHub, builders can quickly prepare data pipelines that be a part of their functions to exterior data sources without having to assemble custom-made integrations from scratch.
LlamaHub will also be open-source, so it’s open to group contributions and new connectors and enhancements are steadily added.
LlamaIndex permits for the creation of superior indexing constructions, similar to vector indexes, and hierarchical or graph-based indexes, to go effectively with varied varieties of data and queries. Vector indexes enable semantic similarity search, hierarchical indexes allow for organized, tree-like layered indexing, whereas graph indexes seize relationships between paperwork or sections, enhancing retrieval for sophisticated, interconnected datasets. These indexing decisions are excellent for functions that should retrieve extraordinarily explicit information or navigate difficult datasets, similar to evaluation databases or document-heavy workflows.
from llama_index.core import VectorStoreIndex, SimpleDirectoryReader
# Load paperwork and assemble index
paperwork = SimpleDirectoryReader("../../path_to_directory").load_data()
index = VectorStoreIndex.from_documents(paperwork)
With LlamaIndex, data may very well be filtered based totally on metadata, like tags, timestamps, or totally different contextual information. This filtering permits actual retrieval, significantly in cases the place data segmentation is required, similar to filtering outcomes by class, recency, or relevance.
from llama_index.core import VectorStoreIndex, Doc
from llama_index.core.retrievers import VectorIndexRetriever
from llama_index.core.vector_stores import MetadataFilters, ExactMatchFilter
# Create paperwork with metadata
doc1 = Doc(textual content material="LlamaIndex introduction.", metadata={"topic": "introduction", "date": "2024-01-01"})
doc2 = Doc(textual content material="Superior indexing methods.", metadata={"topic": "indexing", "date": "2024-01-05"})
doc3 = Doc(textual content material="Using metadata filtering.", metadata={"topic": "metadata", "date": "2024-01-10"})
# Create and assemble an index with paperwork
index = VectorStoreIndex.from_documents([doc1, doc2, doc3])
# Define metadata filters, filter on the ‘date’ metadata column
filters = MetadataFilters(filters=[ExactMatchFilter(key="date", value="2024-01-05")])
# Prepare the vector retriever with the outlined filters
vector_retriever = VectorIndexRetriever(index=index, filters=filters)
# Retrieve nodes
query = "setting pleasant indexing"
vector_nodes = vector_retriever.retrieve(query)
print(f"Vector Outcomes: {[node.text for node in vector_nodes]}")
>>> Vector Outcomes: ['Advanced indexing techniques.']
See one different metadata filtering occasion here.
When to Choose Each Framework
LangChain Fundamental Focus
Difficult Multi-Step Workflows
LangChain’s core energy lies in orchestrating delicate workflows that include plenty of interacting components. Stylish LLM functions sometimes require breaking down difficult duties into manageable steps which may be processed sequentially or in parallel. LangChain affords a robust framework for chaining operations whereas sustaining clear data flow into and error coping with, making it excellent for methods that need to assemble, course of, and synthesize information all through plenty of steps.
Key capabilities:
- LCEL for declarative workflow definition
- Constructed-in error coping with and retry mechanisms
Intensive Agent Capabilities
The agent system in LangChain permits autonomous decision-making in LLM functions. Comparatively than following predetermined paths, brokers dynamically choose from accessible devices and adapt their technique based totally on intermediate outcomes. This makes LangChain notably helpful for functions that should cope with unpredictable client requests or navigate difficult alternative bushes, similar to evaluation assistants or superior buyer help methods.
Widespread agent tools:
Custom tool creation for explicit domains and use-cases
Memory Administration
LangChain’s technique to memory administration solves the issue of sustaining context and state all through interactions. The framework affords delicate memory methods which will monitor dialog historic previous, protect entity relationships, and retailer associated context successfully.
LlamaIndex Fundamental Focus
Superior Info Retrieval
LlamaIndex excels in making big portions of custom-made data accessible to LLMs successfully. The framework affords delicate indexing and retrieval mechanisms that transcend simple vector similarity searches, understanding the development and relationships inside your data. This turns into notably helpful when dealing with big doc collections or technical documentation that require actual retrieval. As an illustration, in dealing with big libraries of financial paperwork, retrieving the suitable information is a ought to.
Key retrieval choices:
- A variety of retrieval strategies (vector, key phrase, hybrid)
- Customizable relevance scoring (measure if query was actually answered by the methods response)
RAG Functions
Whereas LangChain may very well be very succesful for RAG pipelines, LlamaIndex moreover affords a whole suite of devices significantly designed for Retrieval-Augmented Period functions. The framework handles difficult duties of doc processing, chunking, and retrieval optimization, allowing builders to present consideration to establishing functions comparatively than managing RAG implementation particulars.
RAG optimizations:
- Superior chunking strategies
- Context window administration
- Response synthesis methods
- Reranking
Making the Choice
The selection between frameworks sometimes depends upon your software program’s main complexity:
- Choose LangChain when your focus is on target of orchestration, agent conduct, and complicated workflows
- Choose LlamaIndex when your priority is data group, retrieval, and RAG implementation
- Consider using every frameworks collectively for functions requiring every delicate workflows and superior data coping with
It’s additionally important to remember, in a number of cases, each of these frameworks will probably be able to full your job. They each have their strengths, nevertheless for major use-cases similar to a naive RAG workflow, each LangChain or LlamaIndex will do the job. In some cases, the first determining concern is more likely to be which framework you’re most comfortable working with.
Can I Use Every Collectively?
Certain, you presumably can definitely use every LangChain and LlamaIndex collectively. This combination of frameworks can current a robust foundation for establishing production-ready LLM functions that cope with every course of and data complexity efficiently. By integrating the two frameworks, you presumably can leverage the strengths of each and create delicate functions that seamlessly index, retrieve, and work along with in depth information in response to client queries.
An occasion of this integration might presumably be wrapping LlamaIndex efficiency like indexing or retrieval inside a custom-made LangChain agent. This is ready to capitalize on the indexing or retrieval strengths of LlamaIndex, with the orchestration and agentic strengths of LangChain.
Summary Desk:
Conclusion
Deciding on between LangChain and LlamaIndex depends upon aligning each framework’s strengths collectively together with your software program’s desires. LangChain excels at orchestrating difficult workflows and agent conduct, making it excellent for dynamic, context-aware functions with multi-step processes. LlamaIndex, within the meantime, is optimized for data coping with, indexing, and retrieval, good for functions requiring actual entry to structured and unstructured data, similar to RAG pipelines.
For process-driven workflows, LangChain might be going the perfect match, whereas LlamaIndex is sweet for superior data retrieval methods. Combining every frameworks can current a robust foundation for functions needing delicate workflows and durable data coping with, streamlining progress and enhancing AI choices.